Belajar Android Studio dengan Membuat Aplikasi Android Sederhana
Setelah tahapan instalasi selesai dilakukan, kita lanjut dengan membuat aplikasi sederhana dengan Android Studio.
Dalam belajar sesuatu yang baru biasanya saya belajar dari hal yang
mudah-mudah dulu. Ibarat belajar matematika kia tahu angka dulu baru
belajar pertambahan, pengurangan, perkalian dan pembagian. Coba
bayangkan bisakah kita memahami penambahan dalam matematika kalau tidak
tahu angka. Begitu juga dalam pembuatan aplikasi bisakah kita buat
aplikasi Android yang kompleks dan banyak fitur kalau membuat aplikasi
yang sederhana saja tidak bisa.
Aplikasi Android sederhana yang akan kita buat adalah aplikasi yang dapat membantu kita menghitung luas persegi panjang.
Sekarang mari kita mulai
1. Buka Android Studio lalu pilih
Start a new Android Studio Project.

Akan tampil jendela
Create New Project, isikan seperti di bawah ini.

Klik
Next lalau centang
Phone and Tablet

Klik Next, lalu pilih
Blank Activity
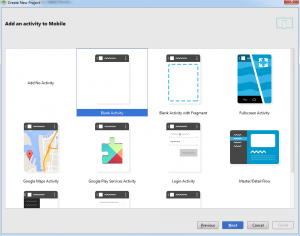
Klik
Next, dan isi Activity Name dan lainnya seperti dibawah ini.

Setelah itu Klik
Finish. Maka secara otomatis menampilkan project yang kita buat.
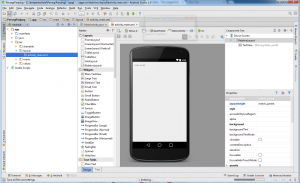
Disini langsung diperlihatkan layout tampilan
activity_main.xml. Dan bisa kita langsung run.
Jika kita punya
Device Android silahkan connectkan ke komputer melalui
usb port.
Klik Tombol Run maka akan muncul pilihan aplikasi uang dirun dimana.
Karena saya sudah menghubungkan Android Moto G saya ke komputer, maka
Moto G saya akan muncul Jendela
Choose Device. Agar lebih cepat runnya Kita pilih saja
Choose Running Device.
Jika tidak punya device maka bisa pilih “
Launch Emulator” kemidan klik OK

Maka hasil tampilannya adalah seperti ini.

Loh aplikasi persegi panjang kok isinya Hello World. Harusnya kan ada inputan panjang dan lebar.
2. Untuk menambahkan inputan panjang dan lebar maka kita harus mengedit file layoutnya dalam hal ini
activity_main.xml untuk itu replace kode yang ada pada file tersebut dengan kode dibawah ini.
03 | android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" |
04 | android:paddingRight="@dimen/activity_horizontal_margin" |
05 | android:paddingTop="@dimen/activity_vertical_margin" |
06 | android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" |
07 | android:id="@+id/relativeLayout"> |
10 | android:layout_width = "wrap_content" |
11 | android:layout_height = "wrap_content" |
12 | android:text = "Panjang" |
13 | android:id = "@+id/textView" |
14 | android:layout_alignParentTop = "true" |
15 | android:layout_alignParentLeft = "true" |
16 | android:layout_alignParentStart = "true" /> |
19 | android:layout_width = "wrap_content" |
20 | android:layout_height = "wrap_content" |
21 | android:inputType = "number" |
23 | android:id = "@+id/editTextPanjang" |
24 | android:layout_below = "@+id/textView" |
25 | android:layout_alignParentLeft = "true" |
26 | android:layout_alignParentStart = "true" |
30 | android:layout_width = "wrap_content" |
31 | android:layout_height = "wrap_content" |
33 | android:id = "@+id/textView2" |
34 | android:layout_below = "@+id/editTextPanjang" |
35 | android:layout_alignParentLeft = "true" |
36 | android:layout_alignParentStart = "true" /> |
39 | android:layout_width = "wrap_content" |
40 | android:layout_height = "wrap_content" |
41 | android:inputType = "number" |
43 | android:id = "@+id/editTextLebar" |
44 | android:layout_below = "@+id/textView2" |
45 | android:layout_alignParentLeft = "true" |
46 | android:layout_alignParentStart = "true" /> |
49 | android:layout_width = "wrap_content" |
50 | android:layout_height = "wrap_content" |
51 | android:text = "Hitung Luas" |
52 | android:id = "@+id/buttonHitungLuas" |
53 | android:layout_below = "@+id/editTextLebar" |
54 | android:layout_alignParentLeft = "true" |
55 | android:layout_alignParentStart = "true" /> |
58 | android:layout_width = "wrap_content" |
59 | android:layout_height = "wrap_content" |
61 | android:id = "@+id/textView3" |
62 | android:layout_below = "@+id/buttonHitungLuas" |
63 | android:layout_alignParentLeft = "true" |
64 | android:layout_alignParentStart = "true" /> |
67 | android:layout_width = "wrap_content" |
68 | android:layout_height = "wrap_content" |
69 | android:inputType = "number" |
71 | android:id = "@+id/editTextLuas" |
72 | android:layout_below = "@+id/textView3" |
73 | android:layout_alignParentLeft = "true" |
74 | android:layout_alignParentStart = "true" /> |
Nah salah satu android studio ini adalah kita bisa langsung lihat previewnya
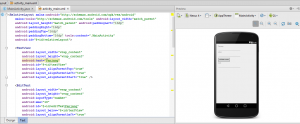
3. Setelah layout selesai sekarang mari kita kerjakan otak dari aplikasi ini yaitu bagian javanya. Buka
MainActivity.java lalu ketikan kode berikut.
01 | package net.agusharyanto.persegipanjang; |
03 | import android.support.v7.app.ActionBarActivity; |
04 | import android.os.Bundle; |
05 | import android.view.Menu; |
06 | import android.view.MenuItem; |
07 | import android.view.View; |
08 | import android.widget.Button; |
09 | import android.widget.EditText; |
11 | public class MainActivity extends ActionBarActivity { |
13 | private EditText edtPanjang; |
14 | private EditText edtLebar; |
15 | private EditText edtLuas; |
16 | private Button btnHitungLuas; |
18 | protected void onCreate(Bundle savedInstanceState) { |
19 | super .onCreate(savedInstanceState); |
20 | setContentView(R.layout.activity_main); |
25 | private void initUI(){ |
26 | edtPanjang = (EditText) findViewById(R.id.editTextPanjang); |
27 | edtLebar = (EditText) findViewById(R.id.editTextLebar); |
28 | edtLuas = (EditText) findViewById(R.id.editTextLuas); |
29 | btnHitungLuas = (Button) findViewById(R.id.buttonHitungLuas); |
32 | private void initEvent(){ |
33 | btnHitungLuas.setOnClickListener( new View.OnClickListener() { |
35 | public void onClick(View v) { |
41 | private void hitungLuas(){ |
42 | int panjang = Integer.parseInt(edtPanjang.getText().toString()); |
43 | int lebar = Integer.parseInt(edtLebar.getText().toString()); |
44 | int luas = panjang*lebar; |
45 | edtLuas.setText(luas+ "" ); |
49 | public boolean onCreateOptionsMenu(Menu menu) { |
51 | getMenuInflater().inflate(R.menu.menu_main, menu); |
56 | public boolean onOptionsItemSelected(MenuItem item) { |
60 | int id = item.getItemId(); |
63 | if (id == R.id.action_settings) { |
67 | return super .onOptionsItemSelected(item); |
4. Kalau dulu di eclipse untuk konfigurasi aplikasi ada difile
AndroidManifest.xml, kalau di Android Studio konfigurasinya ada pada file
build.gradle (module:app)
01 | apply plugin: 'com.android.application' |
05 | buildToolsVersion "21.1.2" |
08 | applicationId "net.agusharyanto.persegipanjang" |
17 | proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' |
23 | compile fileTree(dir: 'libs', include: ['*.jar']) |
24 | compile 'com.android.support:appcompat-v7:21.0.3' |
6.Sekarang mari kita run aplikasi kita. Saya sarankan kita punya
Handphone Android jadi kita bisa langsung Run ke HP. Karena kalau ke Run
menggunakan Emulator itu lambat dan banyak makan Resource komputer kita
Hasil dari aplikasi kita
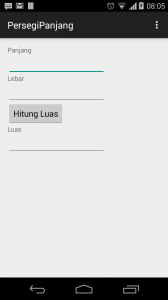
Isikan nilai Panjang dan Lebar kemudian sentuh tombol Hitung Luas
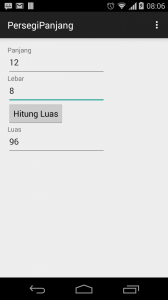
Mudahkan buat aplikasi android dengan android studio.
sumber : http://agusharyanto.net/wordpress/?p=1269